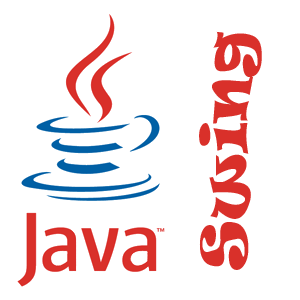
A container object is a
component that can contain other Swing components. A container is responsible
for laying the components that it contains. Commonly used containers are JFrame, JPanel and JScrollPane.
A component is placed on a
user interface and can be made visible or resized. The commonly used components
are JLabel, JTextField, JTextArea, JButton, JCheckBox, JRadioButton and JComboBox.
Java Program to demonstrate simple Swing
import javax.swing.*; public class swg2 extends JFrame { public swg2() { setTitle("my swing application"); setSize(300, 200); setDefaultCloseOperation(EXIT_ON_CLOSE); } public static void main(String args[]) { swg2 s=new swg2(); s.setVisible(true); } }
Java Swing Program to demonstrate GUI Controls [JTextField, JLabel and JButton]
import javax.swing.*; import java.applet.*; import java.awt.*; /*<applet code=swingApp width=300 height=300> </applet> */ public class swingApp extends JApplet { JLabel l1,l2; JTextField t1, t2; JButton b1, b2; public void init() { l1=new JLabel("Enter name"); l2=new JLabel("Enter Email"); t1=new JTextField(20); t2=new JTextField(20); b1=new JButton("OK"); b2=new JButton("Cancel"); setLayout(new FlowLayout()); add(l1); add(t1);add(l2); add(t2); add(b1); add(b2); } }
Java Program to show system time using "util" package
import java.util.*; class Systime { public static void main(String args[]) { Date d=new Date(); System.out.println(d.getHours() + ":"+ d.getMinutes() + ":" + d.getSeconds()); } }
No comments:
Post a Comment